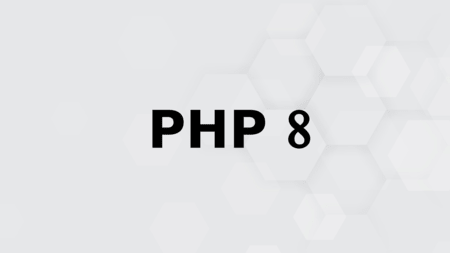
PHP is one of the most widely used programming languages in the world and is used by both small websites and tech giants such as Facebook. The most successful content management systems such as Drupal and WordPress are also based on PHP. The language, which was created by Rasmus Lerdorf, first appeared in 1995 and major versions have been published every three years since then. Version PHP 8.0 was released on 26.11.2020, following on from version 7.4. In this article, you will learn everything you need to know about the new version.
Caution when switching
Before you update your project to version 8, you should carry out a thorough check of your code base. Functions that were marked as deprecated in PHP 7 are no longer supported in PHP 8 and can cause errors if they are not removed. A list of deprecated functions in PHP 7.4 can be found here.
JIT (Just-In-Time) compiler
The Just-In-Time compiler (JIT) is probably the most anticipated new feature in PHP. To understand what the JIT is and what benefits it brings, you first need to understand how PHP code is executed. Put simply, the code is passed to the so-called Zend Engine, which generates machine-readable code from it in several steps. This process must be carried out each time the PHP code is executed and can therefore be quite time-consuming.
With the JIT compiler, there is now a further intermediate step in which the code does not pass through the Zend Engine in several intermediate steps, but is compiled directly into machine-readable code. However, there would be no increase in performance if the entire source code were compiled. The JIT must therefore decide for itself which parts of the source code should be compiled and which should not.
As already mentioned, the great advantage of a JIT is that it can significantly improve performance. However, the extent of the impact ultimately depends on the type of program being executed. With conventional web applications, the performance boost is rather small, as a large part of the application logic is occupied with database queries, on whose speed the JIT compiler has no influence. Larger improvements are more likely to be seen in other areas that are not normally immediately associated with PHP, such as machine learning. This can therefore help to establish PHP as a general-purpose language, i.e. a programming language that can also be used outside the web.
Constructor property promotion
Anyone who has ever written object-oriented PHP knows the following inconvenience:
class Person {
public string $name;
public string $address;
public function __construct(
string $name,
string $address,
) {
$this->name = $name;
$this->address = $address;
}
}
That's a lot of boilerplate code to fill the properties of the class. Especially if there are several properties, this can be a real time waster and make the code unnecessarily long. In version 8.0, it is now possible to declare the properties directly in the constructor:
class Person {
public function __construct(
public string $name,
public string $address,
) {}
}
This notation leads to the same result as the previous one, only with much less code. Instead of first defining the properties and then filling them with the passed arguments within the constructor, the properties including the access modifier are declared and filled directly in the arguments of the constructor. The function body can then even remain empty - PHP knows what to do! If you would like more information about object-oriented programming in PHP, we have the right article for you in our blog: Object-oriented programming: explained using Drupal as an example.
Typing
Types in PHP have come a long way. PHP is "weakly typed", which means that typing is not necessary for variables and classes. For example, it is not necessary to specify whether a variable is a string or a number. Although this simplifies the writing of code, it can lead to unforeseen bugs over time, which could have been avoided with more type safety. Therefore, PHP 5 introduced the (non-mandatory) option of declaring types, with the list of available types growing over time.
Union Types
One problem with types in PHP used to be that only a single type could be specified in functions. For example like this:
function myFunction (string $name): string {
// Some logic.....
}
This code means that the argument "$name" of type string must be passed to the function and the functions returns a string. But what if a function only returns a string if everything goes well and "false" if something goes wrong, e.g. if a sanity check fails? This case was previously not covered and you had to ensure that a string was always returned - or that the return type was not specified at all.
This is now different with PHP 8. The following syntax is now possible:
function myFunction (string|boolean|date $name): string|false {
// Some logic...
}
There are several anomalies here:
- The argument "$name" can now be either of type String, Boolean or Date. The different possibilities are separated by a pipe ("|").
- The return type is now "string|false". This means that either a string or false is returned. You could just as well have written "string|boolean", but "false" is even more descriptive.
"Mixed" as return value
In PHP 8, the return value "mixed" is now also supported. This makes the following possible:
function myFunc (): mixed {
// Some logic...
}
The "mixed" type causes discussions across programming languages. After all, what is the point of (strong) typing if you simply bypass it? Then you might as well leave out the return value, right? Not quite. Because a missing specification of the return value can mean many things, e.g. that nothing is returned, that the return type cannot be displayed in PHP or that it can be one of many different return values. By specifying "mixed", it is clearly indicated that this is the last of the three cases.
"Static" as return value
Previously, it was possible to specify "self" as the return value of a function. Now it is also possible to use "static" as the return value:
class MyClass {
public function myFunc(): static {
return new static();
}
}
Named arguments
Named arguments allow the programmer to pass arguments to a function by name rather than position. This looks like this:
function myFunc ($name, $address) {
// Some logic...
}
myFunc(address: 'Wayne Manor', name:'Bruce Wayne')
Although "$address" is in second place in the arguments in the definition of the function, the address can be passed first when the function is called by marking the argument with "address:". This means that the programmer does not have to remember the order in which the arguments are to be specified and this notation is also more descriptive.
"Throw" as an expression, not as a statement
Previously, "throw" could only be used as a statement. As of PHP 8, this has been changed so that "throw" is now an expression. This enables a variety of new places where exceptions can now be thrown. For example:
$my_var = $variables['some_index'] ?? throw new Error();
New functions
Of course, PHP 8 also comes with some new functions that make life easier for the programmer. The most important new features relate to working with strings.
str_contains()
Until now, you had to take a small detour via the "strpos" function to find out whether a character string contains another character string. There is now a separate function for this:
str_contains('This is my string and it is very cool.', 'cool') // TRUE
str_starts_with() and str_ends_with()
Two other functions that deal with strings are str_starts_with() and str_ends_with(). As the names suggest, they can be used to check whether a character string starts or ends with another character string:
str_starts_with('haystack', 'hay'); // TRUE
str_ends_with('haystack', 'stack'); // TRUE
get_debug_type()
This function can be used to return the type of a variable. This could also previously be achieved using the "gettype()" function. However, "get_debug_type()" returns more detailed information. For example, "gettype()" would return the class MyClass "object" while "get_debug_type()" would return the name of the class.
Drupal Support
PHP 8 is currently supported by Drupal 7 (from 7.79) and 9.1. Drupal 8 and 9.0, however, do not work with the new PHP main version. But beware: Community support for PHP 7.4, the latest PHP version to date, will end at the end of 2021. There will then be no more updates and no more security gaps will be closed. Then you should not only consider a PHP update, but also a Drupal 9 upgrade. As an agency specializing in Drupal, we are happy to support you! You can write to us here at any time or call us directly.
Summary
With PHP 8, a new major version has been released that brings with it many new features. In particular, the eagerly awaited just-in-time compiler is set to take the programming language to the next level. But other new features such as named arguments or new types also improve the experience for the programmer.